Project BBEG Blog #2: Dungeon Generation refinement & Artifacts system implementation challenges
- Dan Hartman
- May 18, 2023
- 3 min read
We're getting closer and closer to a playable prototype of Project BBEG. Today I'll be sharing some progress made on dungeon generation and an early version of our character progression system, which I'll refer to from now on as the Artifacts system.
First, the dungeon generation updates. Previously, dungeon generation only accounted for a single square room size. Anything larger would start overlapping with other rooms. To remedy this, each room now has an all-encompassing box collider attached on a layer called RoomExtents. New room spawns check to see if they overlap with other rooms by using an Physics.OverlapBox() call to search for colliders on the RoomExtents layer.
Thanks to this change, our rooms can have different sizes and orientations without overlapping, leading to results looking like the image below.
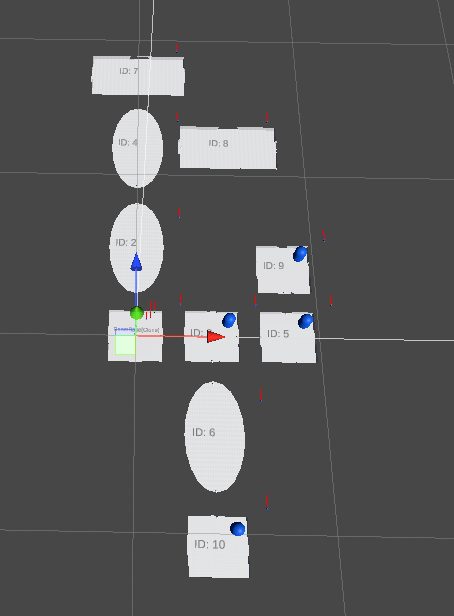
Up next is Artifacts. Artifacts range from simple stat boosts such as higher ranged attack damage, to items with ArtifactBehaviors such as dealing extra damage when HP is low. Sometimes, Artifacts will have both of these, so I decided to start by working on Buffs and ArtifactBehaviors mostly separate, and have Artifacts simply contain one of each.
The first step I took when implementing this was to create a buffs system that allows for stat changes without directly modifying a character's base stats.
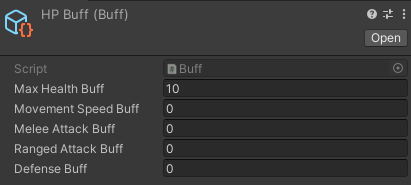
Characters now have a list of buffs and a helper function for each major stat to add their base stats and buffs stats together. This way, buffs can easily be added or removed without any issues. Additionally, buffs can be given to enemies as well since they inherit from the Character class. Here's the max health function for example:
public int GetMaxHealth()
{
int maxHealthBuffTotal = 0;
foreach(Buff b in activeBuffs)
{
maxHealthBuffTotal += b.maxHealthBuff;
}
return maxHealthBuffTotal + baseHealth;
}
ArtifactBehaviors were a bit more difficult to implement, and have some issues that need to be addressed before I can consider it a complete feature. My original vision was for the Artifact to contain a reference to a component, and use AddComponent to add it to ArtifactManager's component list. However, two problems came up during implementation.
public class LowHPAttackBoost : ArtifactBehaviorBase
{
public float healthPercentageRequirement = .2f;
public float damageModifier = 1.2f;
void ApplyAttackBoost(HitData hit)
{
float healthPercentage = hit.mOwner.currHealth / hit.mOwner.GetMaxHealth();
if(healthPercentage < healthPercentageRequirement)
{
hit.mDamage *= damageModifier;
}
}
// Start is called before the first frame update
void Start()
{
EventManager.instance.onAttackConnected += ApplyAttackBoost;
}
private void OnDestroy()
{
EventManager.instance.onAttackConnected -= ApplyAttackBoost;
}
}
This code works, but because I'm adding these components in during runtime I can't simply change their variables in the editor beforehand. For now I can only change their default values in the code directly. I plan to remedy this by researching alternate methods of assigning values to variables. I could potentially have it parse a spreadsheet that also includes other data.
The second problem involves the Artifact containing a reference to the ArtifactBehavior component. According to my research at least, this isn't possible in Unity and I have to perform a workaround. What I ended up doing was creating an enum that contains every ArtifactBehavior script, and running a switch statement that manually calls AddComponent for each corresponding script.
switch(artifact.behavior)
{
case ArtifactBehaviorType.LowHPAttackBoost:
{
behaviorComponent = gameObject.AddComponent<LowHPAttackBoost>();
break;
}
// For each new ArtifactBehavior add a new case
}
As I'm writing this, I'm coming to terms with the fact that despite this code working just fine, I should go back to the drawing board and find a better solution for ArtifactBehaviors that addresses these two issues. The above switch statement will quickly get obtuse and messy down the line. The pipeline for designers to edit ArtifactBehaviors also needs to be smooth since there will be so many of them.
If you want to follow the development of Project BBEG, here's a link to our discord server: https://discord.gg/e5M5uwa5UY
Comments