Flow Field pathfinding
- Dan Hartman
- Mar 6, 2022
- 2 min read
To implement flow field pathfinding into my current AI framework, I started fresh in a new scene and made a class called FlowFieldGrid. This class is responsible for creating the grid at runtime and computing the main three steps of flow field pathfinding, those steps being Cost Field, Indication Field, and Flow Field.
Firstly, I decided to make my own grid using Unity's ever so useful gizmos feature. Initially I thought about having FlowFieldGrid handle drawing the grid, but I found that simply having each grid cell draw a box around itself with the proper dimensions was simpler to implement and gave the same results.
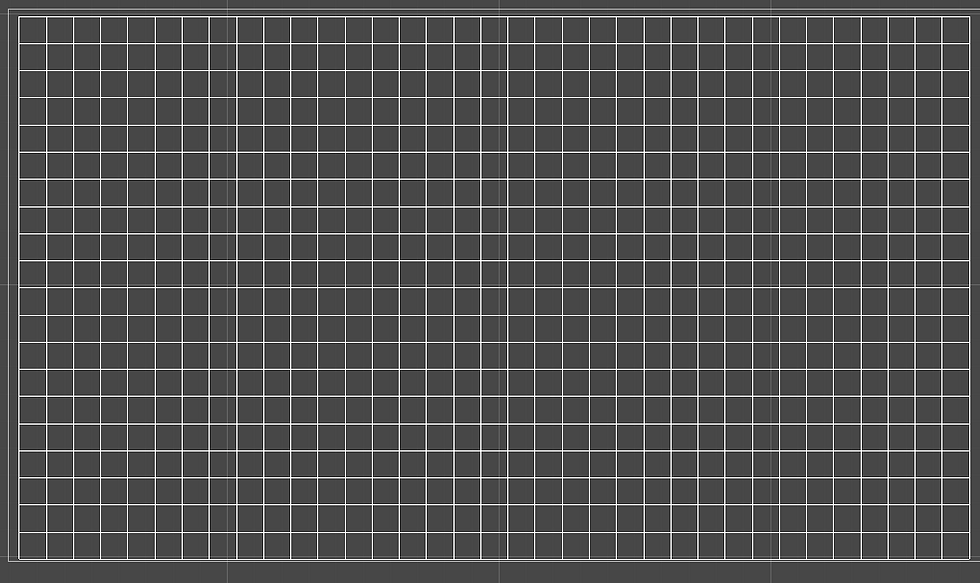
Next, the cost field step is all about checking each grid spot for any impassible or rough terrain. Hard obstacles would be walls, being absolutely impassable, and a soft obstacle is still passable but it's set to a higher cost than an empty cell, to encourage our boids to try other avenues before resorting to traversing through the obstacle. I used a 2D List to represent the grid cells.

The second step of flow field is the indication field. This step's goal is to assign each cell a "best cost", which means the lowest possible total cost required to reach that specific cell starting from the destination cell. It is important to note that due to varying costs that might come from different terrain, this does not necessarily mean lowest cost is always the shortest route.
Yellow circle = Target, Black = impassible, Red = rough terrain.
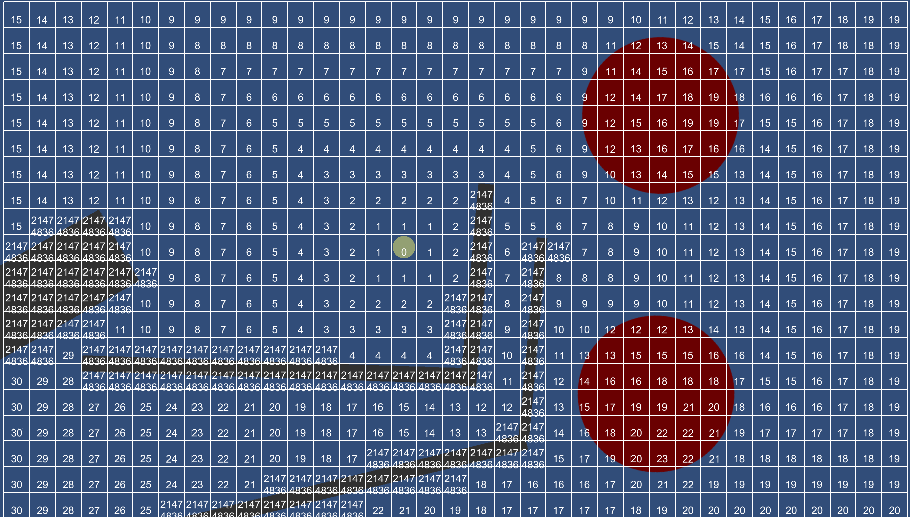
The final step is creating the "flow" that boids can use to move to a destination. This is done by checking the neighboring cells, finding which one has the lowest best cost value, and setting a direction vector towards it, creating a visually pleasing map of arrows, or in this case, Unity gizmos. Then, I assigned each GridCell a direction and made a helper function for boids to be able to tell what cell they are in so they can access the cell's direction value.

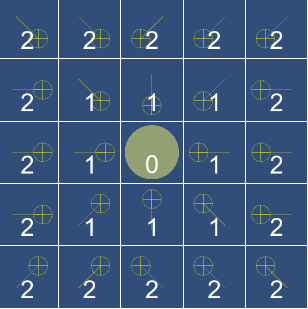
To implement my boids, I created a new type of steering specifically for the flow field scene and set the boids to only run flow field steering with a small amount of separation steering to make it look a bit nicer. Additionally, I have the flow field set to regenerate as often as I'd like to account for moving terrain.

Here it is in action:
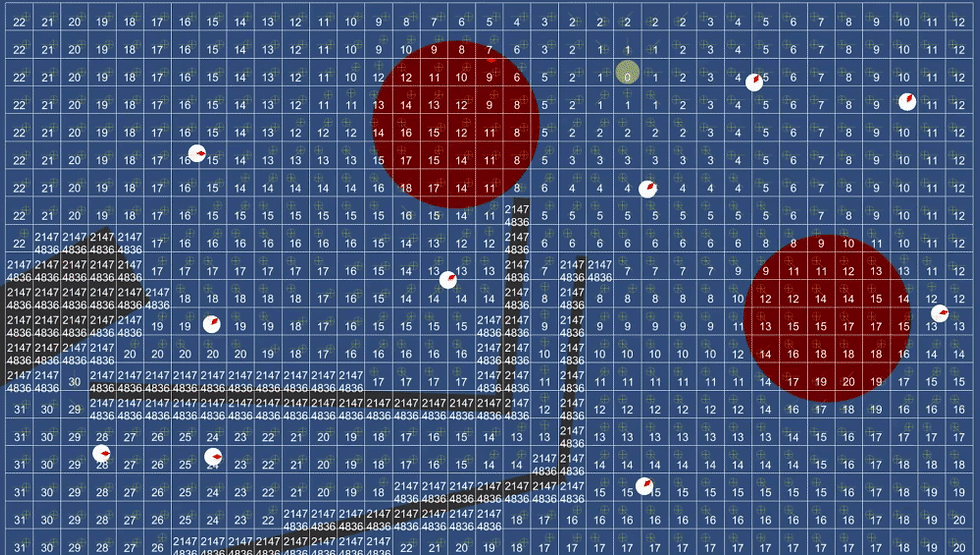
Comentarios